React Chat Integration: A Simple Guide to Adding Chat in React
March 19, 2025
Introduction of React Chat
Chat features are now a must-have in today’s websites and apps. They let people talk to each other in real-time making things more interactive. Whether it’s a messaging app, live customer support, or a team collaboration tool, adding chat to your React app can make it more interactive and engaging.
In this guide, we’ll break down how chat works in React, explore the best libraries to use, and walk through a step-by-step process with simple code examples. Let’s get started! 🚀
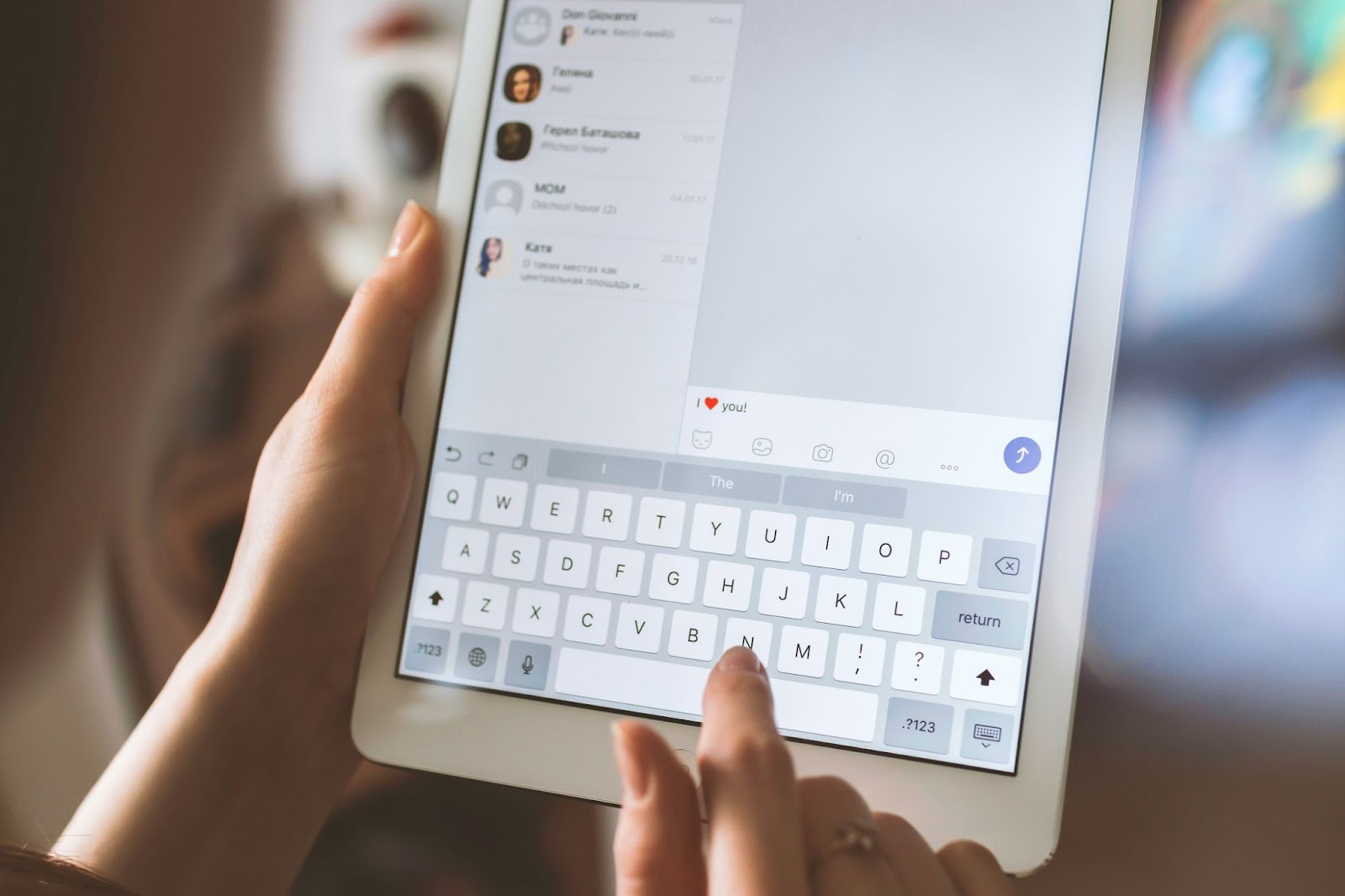
How React Chat Works
For real-time chat, your app needs to constantly exchange messages between the client (your React app) and the server. Here are three popular approaches to accomplish this:
- WebSockets: This creates a constant connection between the client and server, allowing instant back-and-forth communication. It’s the fastest and most efficient method for real-time chat.
- Polling: Here, the app keeps checking the server every few seconds to see if there are new messages. It’s simple but can be slow and use a lot of resources.
- Server-Sent Events (SSE): With this method, the server sends updates to the client automatically, but it’s a one-way communication (server to client only).
Out of these, WebSockets is the best choice for real-time chat apps because it’s fast and works both ways.

Best Libraries for React Chat Integration
Adding chat to your React app is easier with the help of some great tools. Here are the top options:
- Socket.io: A popular library for real-time communication. It uses WebSockets to make chat fast and reliable.
- Stream Chat: A ready-to-use chat solution with lots of features. It’s powered by APIs, so you don’t have to build everything from scratch.
- Firebase Firestore: This is a real-time database that automatically updates when new messages are sent. It’s great for simple chat setups.
- ChatEngine: A complete chat service that handles everything for you. It’s perfect if you want to add chat quickly without much coding.
Step-by-Step Guide to Implementing React Chat
Step 1: Setting Up a React Project ⚙️
To start, you’ll need a React project. You can create one using tools like Vite. Here’s how:
Step 2: Installing Dependencies
For this example, we'll use Socket.io for real-time messaging.
Step 3: Creating the Chat Component (Frontend)
Modify App.js to connect the frontend with a WebSocket server.
Step 4: Running the Application
Run the frontend:
(Minimal Backend Explanation) 🖥️
For real-time chat to work, you need a WebSocket server. This server helps the app send and receive messages instantly. Here’s a quick example of how to set up a simple backend using Express and Socket.io.
This backend simply listens for messages and broadcasts them to all clients. You can set up a local server or use services like Firebase for real-time data handling.
Advanced Features for React Chat
Once your basic chat is working, you can make it even better by adding cool features like:
- User authentication Make sure only logged-in users can chat. You can use tools like Firebase Authentication or JWT (JSON Web Tokens) for this.
- Typing indicators: Real-time typing indicators notify users when someone is actively composing a message. It’s a small touch that makes chat feel more alive.
- Read receipts: Let users know when their messages have been seen by others.
- Media sharing: Allow users to send pictures, videos, or files in the chat.
Security Considerations for React Chat
- Use HTTPS & WSS: Always use secure connections (HTTPS for the website and WSS for WebSockets) to protect data while it’s being sent.
- Add Authentication & Authorization: Make sure only the right people can access the chat. Use tools like Firebase Authentication or JWT to check who’s allowed in.
- Encrypt Messages: Keep messages private by encrypting them. This way, even if someone intercepts them, they can’t read the content.
Conclusion
Adding chat to a React app is now simpler than ever, thanks to tools like Socket.io, Firebase, and Stream Chat. Whether you’re building a customer support chat, a group messaging app, or a real-time notification system, these tools make it easy and scalable.
By following this guide, you should now have a working React chat app. Happy coding! 🚀