useMemo vs useCallback: Understanding the Key Differences in React
March 7, 2025
useMemo vs useCallback: Introduction
When building React applications, performance optimization is a crucial aspect of creating a smooth user experience. Poorly optimized applications can lead to sluggish performance, unnecessary re-renders, and slow user interactions. To combat this, React provides built-in hooks such as useMemo() and useCallback() that help developers enhance performance by avoiding unnecessary computations and rendering cycles.
Understanding these hooks is crucial for writing efficient React applications. While both useMemo and useCallback serve similar performance-enhancing purposes, they function differently and are best suited for distinct scenarios. Using the right hook at the right time can significantly improve the efficiency and responsiveness of your application. In this blog, we will explore the differences between useMemo and useCallback, their use cases, and when to use each effectively.
What is useMemo?
useMemo() is a React hook that memoizes the result of a function to prevent unnecessary calculations. This means that React will store the computed value and only re-compute it when its dependencies change.
Syntax:
Key Points:
- Returns a memoized value: The hook caches the return value of a function to prevent redundant computations.
- Triggered by dependency changes: The function inside useMemo only runs when the dependencies in the array change.
- Optimizes expensive calculations: Commonly used to optimize functions that perform heavy calculations.
Example:
In this example, the function to compute the square of a number will only run when num changes, preventing unnecessary recalculations.
What is useCallback?
useCallback() is a React hook that memoizes a function reference to prevent unnecessary re-creation of functions.
Syntax:
Key Points:
- Returns a memoized function: Unlike useMemo, which caches a computed value, useCallback stores a function reference.
- Useful for passing stable functions as props: Helps prevent unnecessary re-renders when passing functions to child components.
- Triggered by dependency changes: The memoized function will only change if the specified dependencies change.
Example:
In this example, useCallback ensures that the increment function maintains the same reference between renders, preventing unnecessary re-renders of the Button component.
Key Differences Between useMemo and useCallback

When to Use useMemo vs useCallback
Understanding when to use useMemo and useCallback can sometimes be tricky. The flowchart below provides a visual guide to help determine the right hook based on your use case. Follow the decision-making process to
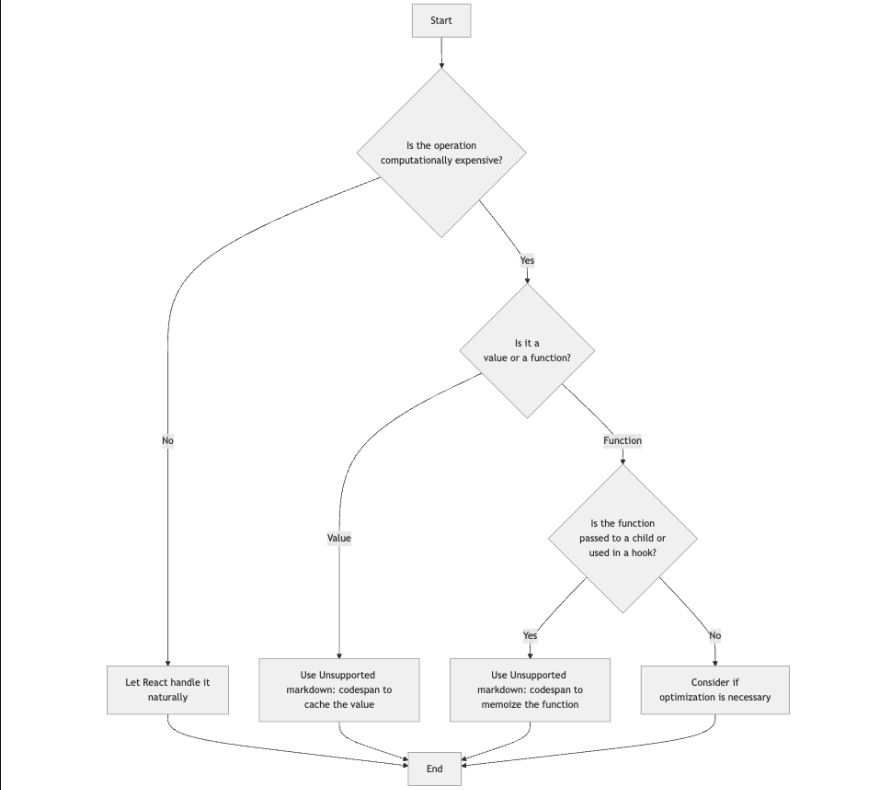
Use useMemo When:
- You need to optimize expensive calculations that would otherwise run on every render.
- You want to cache a computed value to improve performance.
- The computation is dependent on specific values that change infrequently.
Use useCallback When:
- You need to ensure a function reference remains stable between renders.
- You're passing a function as a prop to a child component and want to prevent unnecessary re-renders.
- You want to optimize event handlers in functional components.
Common Mistakes to Avoid
- Overusing useMemo and useCallback:
- Using these hooks unnecessarily can add complexity without significant performance benefits.
- Ignoring Dependencies:
- Always specify the correct dependencies; otherwise, memoization may not work correctly.
- Misusing useMemo Instead of useCallback:
- If you need to memorize a function reference, use useCallback, not useMemo.
useMemo vs useCallback:Conclusion
Understanding when and how to use useMemo and useCallback can greatly improve the performance of your React applications. While useMemo optimizes expensive calculations by memoizing their results, useCallback prevents unnecessary function re-creations, reducing unwanted re-renders. By applying these hooks correctly, you can enhance the efficiency and maintainability of your React components. However, always remember to use them wisely, as unnecessary usage can lead to added complexity without tangible benefits.
By leveraging these hooks effectively, you can build highly performant React applications with smoother user experiences.